How to Resize ProgressView in SwiftUI
Learn how to adjust the size of ProgressView in your SwiftUI app with these helpful tips and tricks
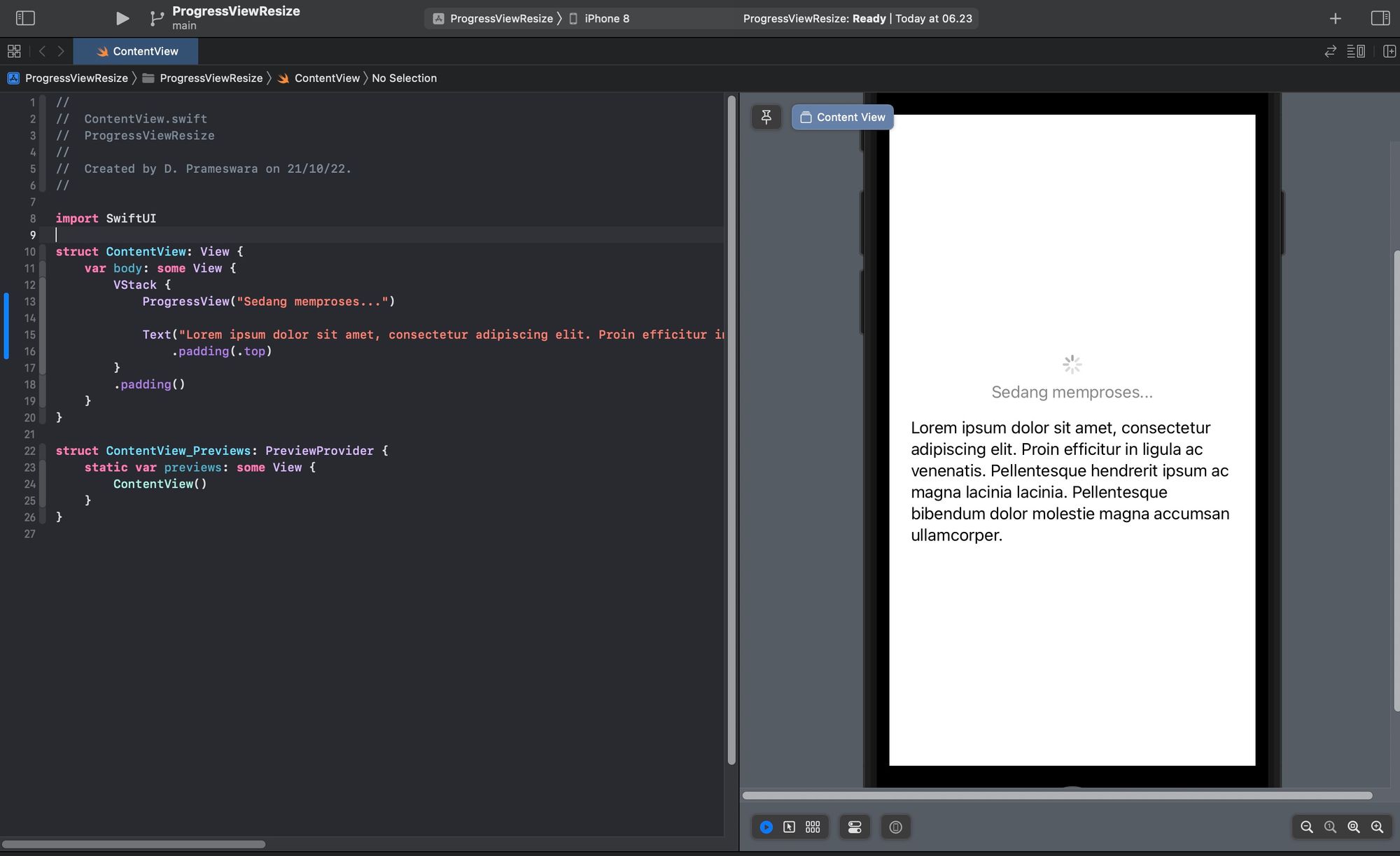
#What is ProgressView? ?
ProgressView is a component in SwiftUI used to display the progress of a process or similar tasks, whether in linear or circular form.
struct ProgressView<Label, CurrentValueLabel> where Label : View, CurrentValueLabel : View
However, ProgressView may appear too small by default, as shown in the image below.
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
ProgressView("Sedang memproses...")
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Proin efficitur in ligula ac venenatis. Pellentesque hendrerit ipsum ac magna lacinia lacinia. Pellentesque bibendum dolor molestie magna accumsan ullamcorper.")
.padding(.top)
}
.padding()
}
}
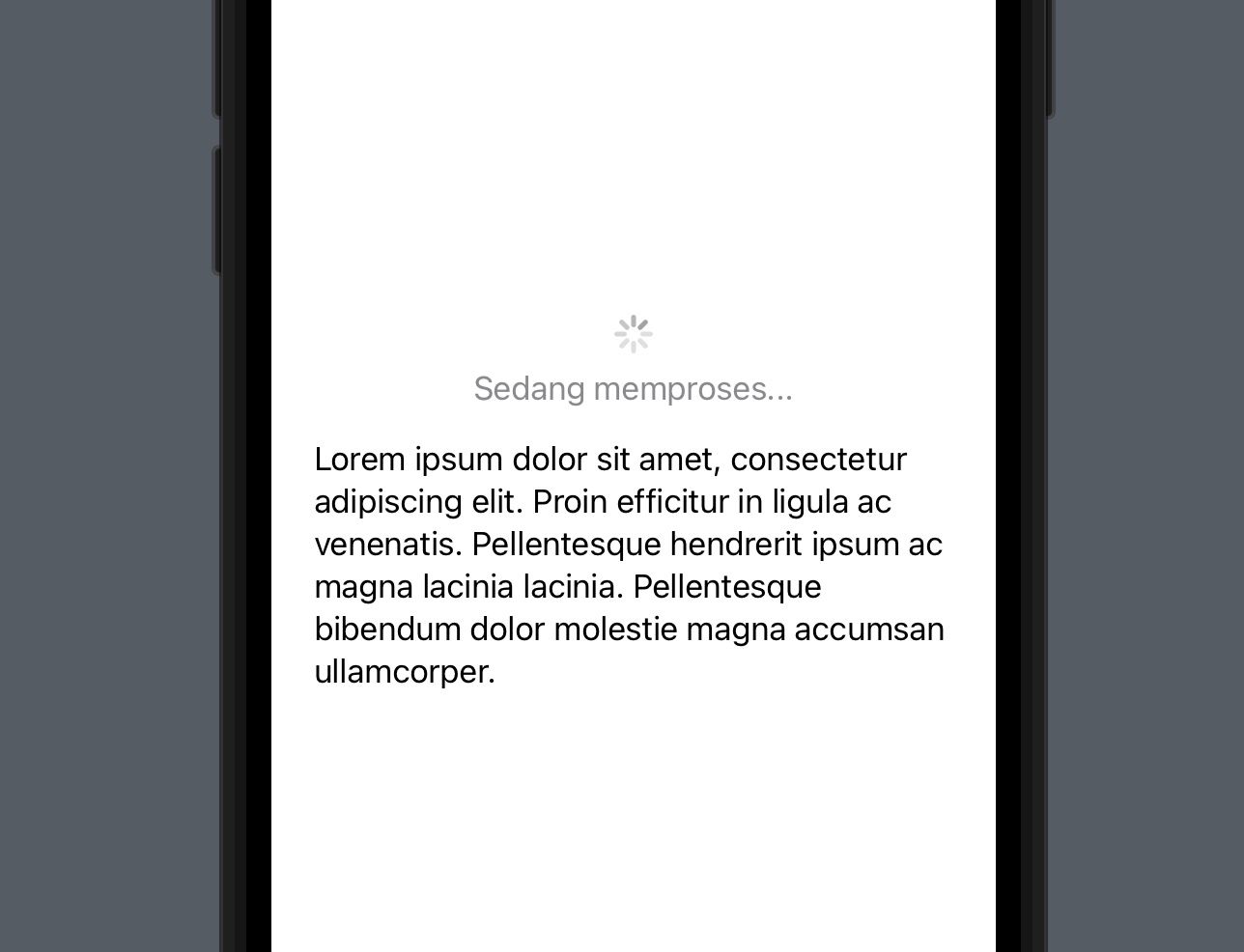
#Resizing ProgressView using scaleEffect
To change the size of ProgressView, you can use the scaleEffect(_ s: CGFloat, anchor: UnitPoint = .center)
modifier. Simply add this modifier with the desired value and anchor point.
struct ContentView: View {
var body: some View {
VStack {
ProgressView("Sedang memproses...")
.scaleEffect(2, anchor: .center)
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Proin efficitur in ligula ac venenatis. Pellentesque hendrerit ipsum ac magna lacinia lacinia. Pellentesque bibendum dolor molestie magna accumsan ullamcorper.")
.padding(.top)
}
.padding()
}
}
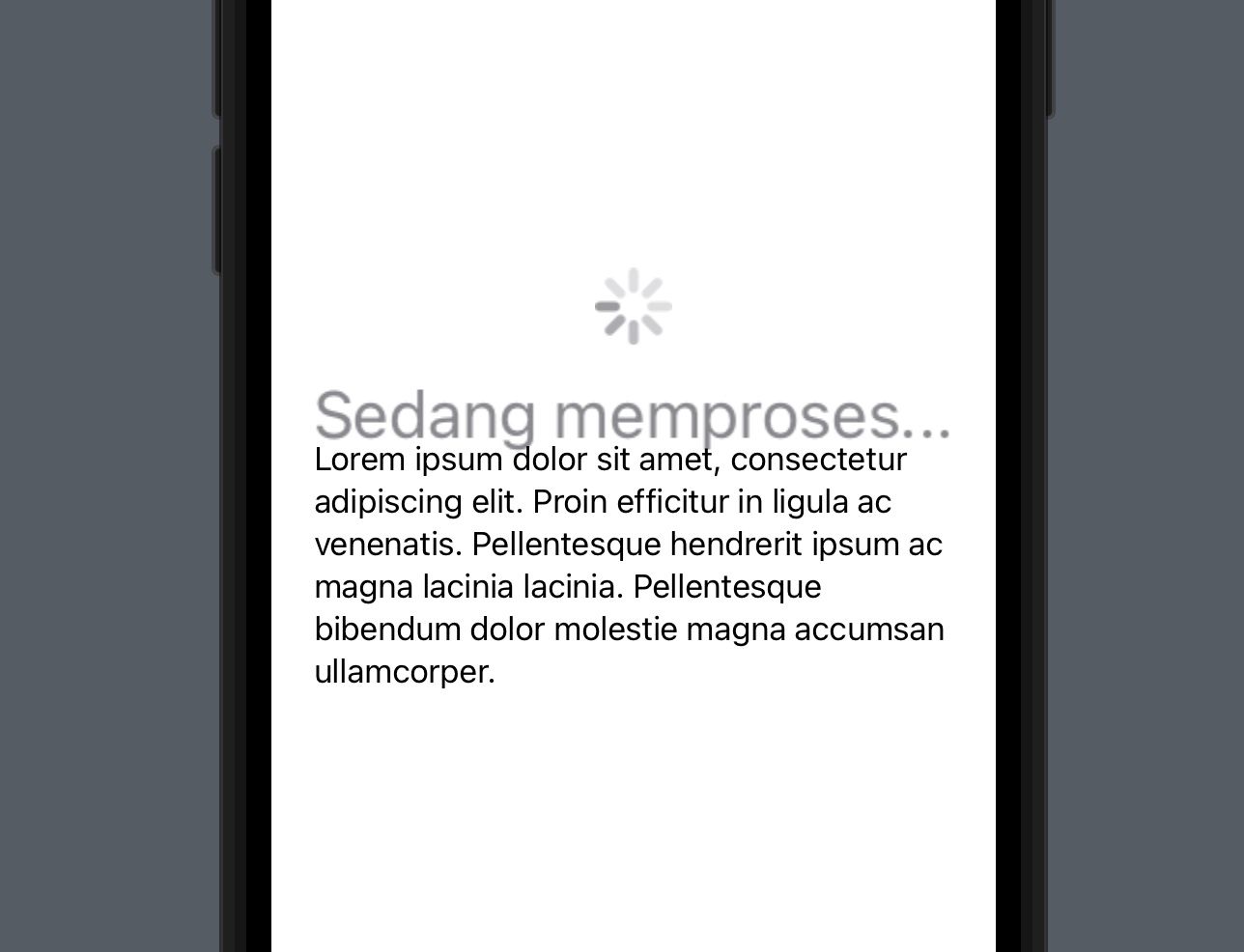
In the image above, not only does the size of ProgressView change, but the label also scales.
#Separating the label from ProgressView
Therefore, if you only want to resize the Progress itself, separate the text/label from your ProgressView as follows.
struct ContentView: View {
var body: some View {
VStack {
ProgressView()
.scaleEffect(2, anchor: .center)
.padding()
Text("Sedang memproses...")
.foregroundColor(.secondary)
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Proin efficitur in ligula ac venenatis. Pellentesque hendrerit ipsum ac magna lacinia lacinia. Pellentesque bibendum dolor molestie magna accumsan ullamcorper.")
.padding(.top)
}
.padding()
}
}
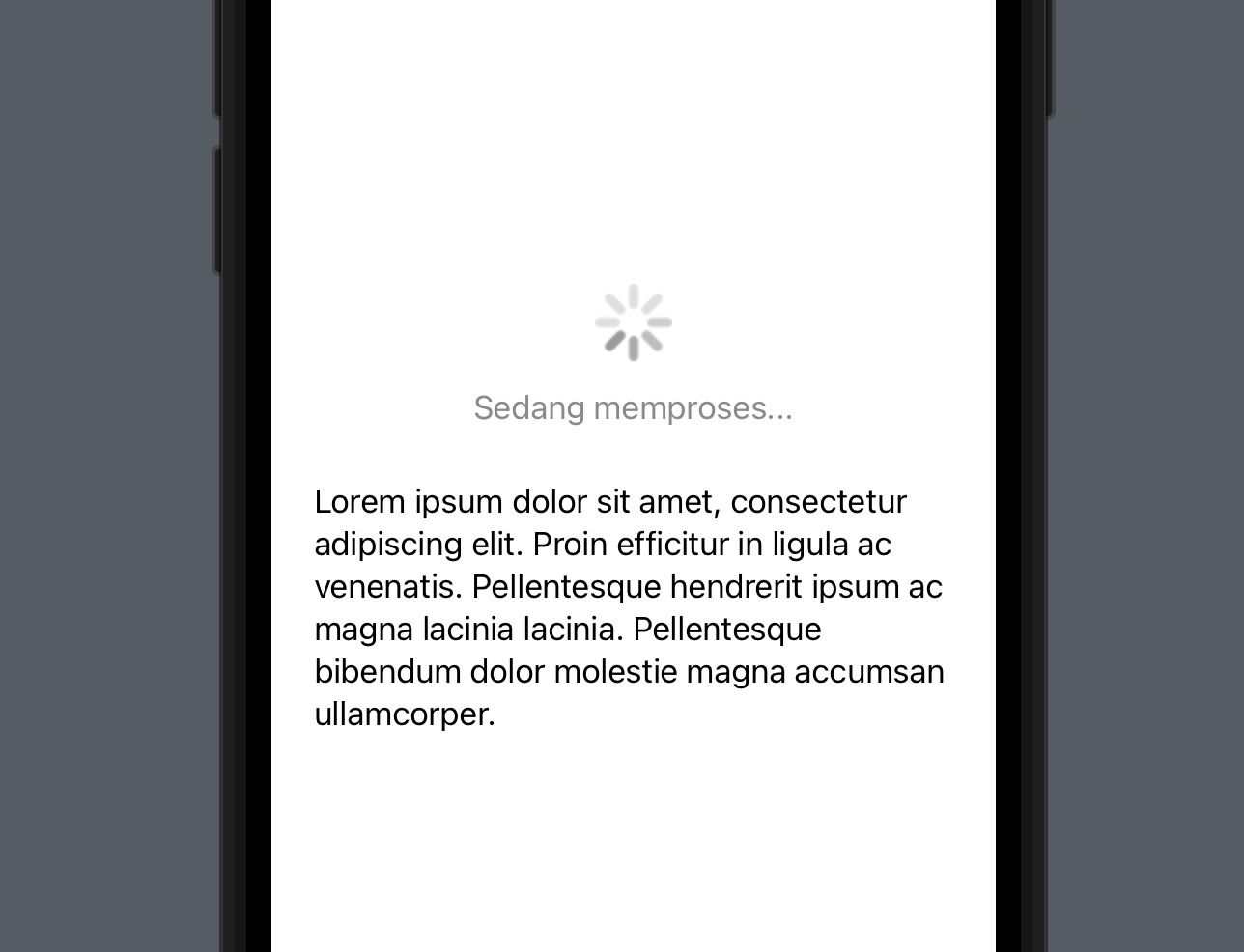
#Conclusion
The size of ProgressView can be adjusted using the scaleEffect
modifier. However, keep in mind that this modifier not only scales the ProgressView but also the label within it. Additionally, excessively large scaleEffect
values can distort the appearance of ProgressView, making it appear blurry. Therefore, choose an appropriate scale value that is not too large.