Creating Round Corners on Specific Corners of a View
Learn how to customize your SwiftUI views with specific rounded corners
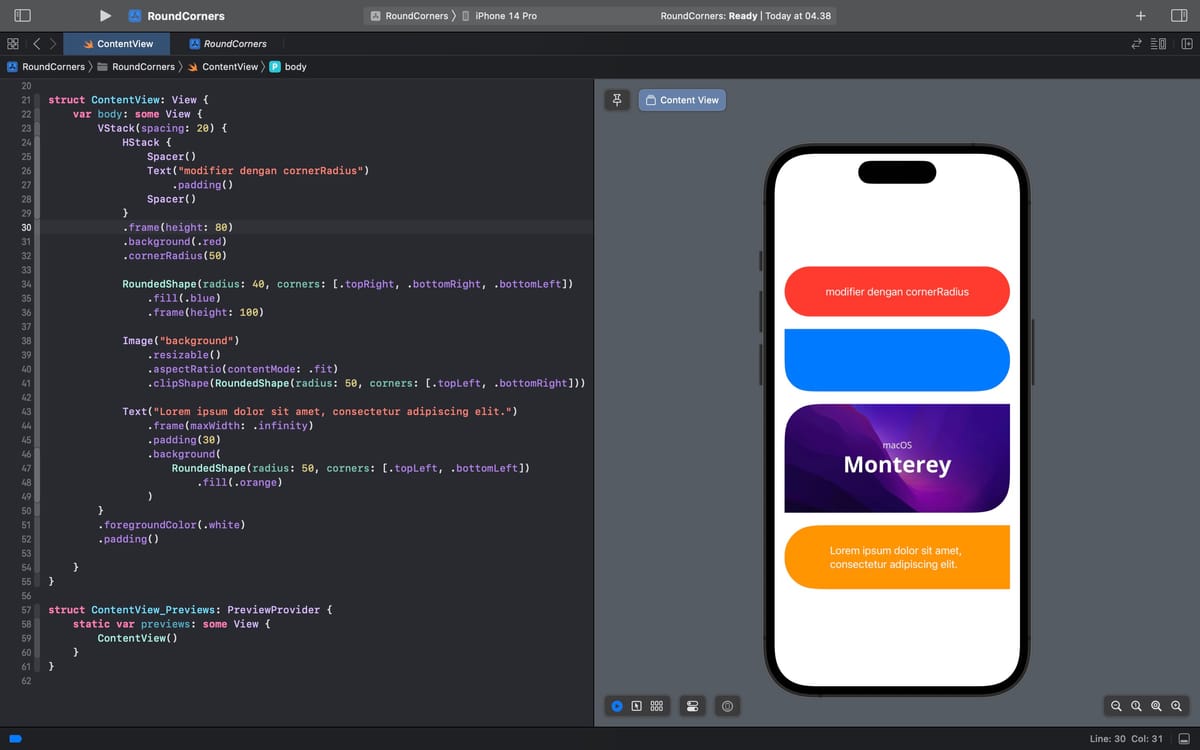
In SwiftUI, when you want to give a view rounded corners, you can use the built-in cornerRadius
modifier with a specified radius like this:
func cornerRadius(
_ radius: CGFloat,
antialiased: Bool = true
) -> some View
cornerRadius
modifier
Here's an example of how to use the cornerRadius
modifier:
struct ContentView: View {
var body: some View {
VStack {
HStack {
Spacer()
}
.frame(height: 80)
.background(.red)
.cornerRadius(50)
}
.padding(30)
}
}
Using the cornerRadius
modifier
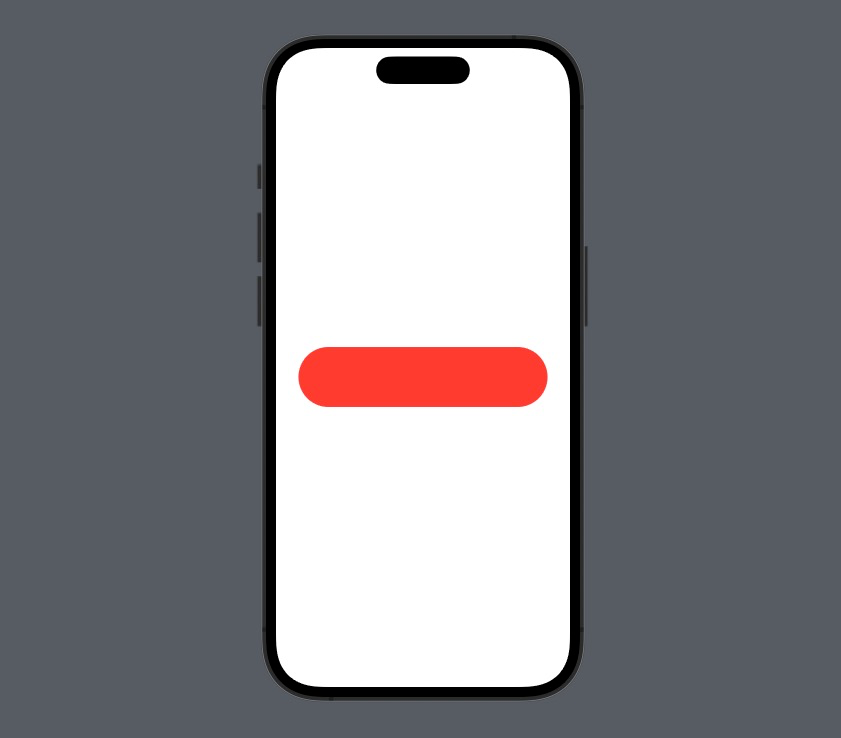
However, using the cornerRadius
modifier will make all corners rounded, which may not always be the desired effect. Sometimes, you might want only specific corners to be rounded. To achieve this, you need to use other techniques like the clipShape
or background
modifiers. Both of these modifiers require a shape to determine the corners and radius.
#Creating a Shape to Control Corners and Radius
The first step is to create a custom shape that allows you to specify the radius and corners. Let's call this shape "RoundedShape":
struct RoundedShape: Shape {
var radius = CGFloat.infinity
var corners = UIRectCorner.allCorners
func path(in rect: CGRect) -> Path {
let path = UIBezierPath(
roundedRect: rect,
byRoundingCorners: corners,
cornerRadii: CGSize(width: radius, height: radius)
)
return Path(path.cgPath)
}
}
RoundedShape
The custom shape above can be called by setting the radius and corners properties. The radius determines how large the corner radius is, while corners specifies which corners have their radius defined. The corners value can be filled with topLeft, topRight, bottomLeft, bottomRight, and allCorners.
You can use this custom shape by specifying the radius and the corners :
struct ContentView: View {
var body: some View {
VStack {
RoundedShape(radius: 40, corners: .bottomRight)
.fill(.red)
.frame(height: 100)
.padding()
RoundedShape(radius: 40, corners: .topLeft)
.fill(.blue)
.frame(height: 100)
.padding()
RoundedShape(radius: 40, corners: [
.topRight, .bottomRight, .bottomLeft
])
.fill(.orange)
.frame(height: 100)
.padding()
}
}
}
Using the custom shape
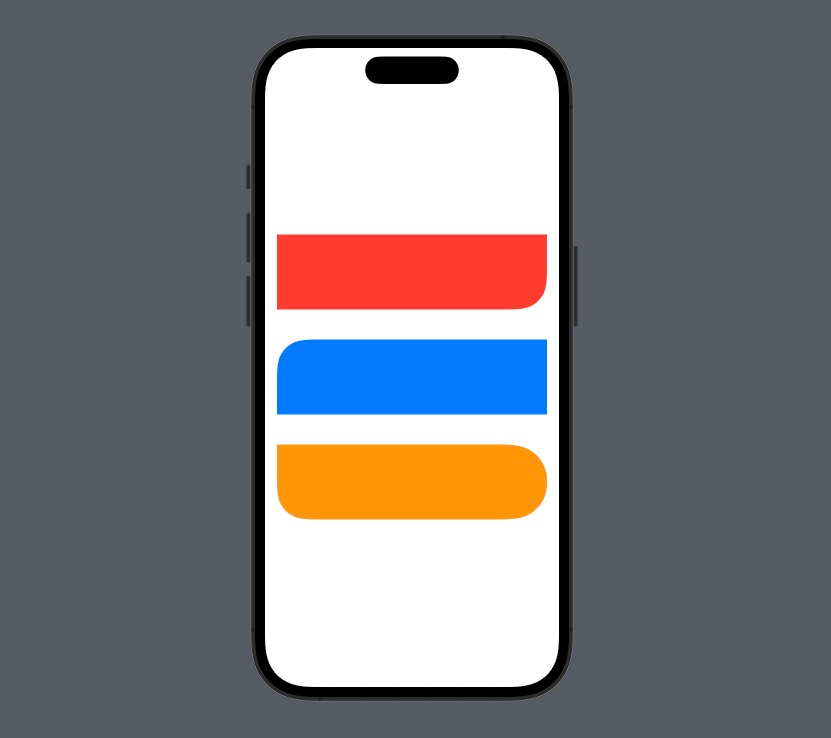
#Controlling Round Corners with clipShape
By using the custom shape created above, you can control the radius and specific corners of a view by using the clipShape
modifier:
func clipShape<S>(
_ shape: S,
style: FillStyle = FillStyle()
) -> some View where S : Shape
clipShape modifier
This modifier is typically used to create a mask on a view, such as an image or a button:
struct ContentView: View {
var body: some View {
VStack {
Image("background")
.resizable()
.aspectRatio(contentMode: .fit)
.clipShape(
RoundedShape(
radius: 50,
corners: [.topLeft, .bottomRight]
)
)
}
.padding(30)
}
}
Example of using the clipShape
modifier
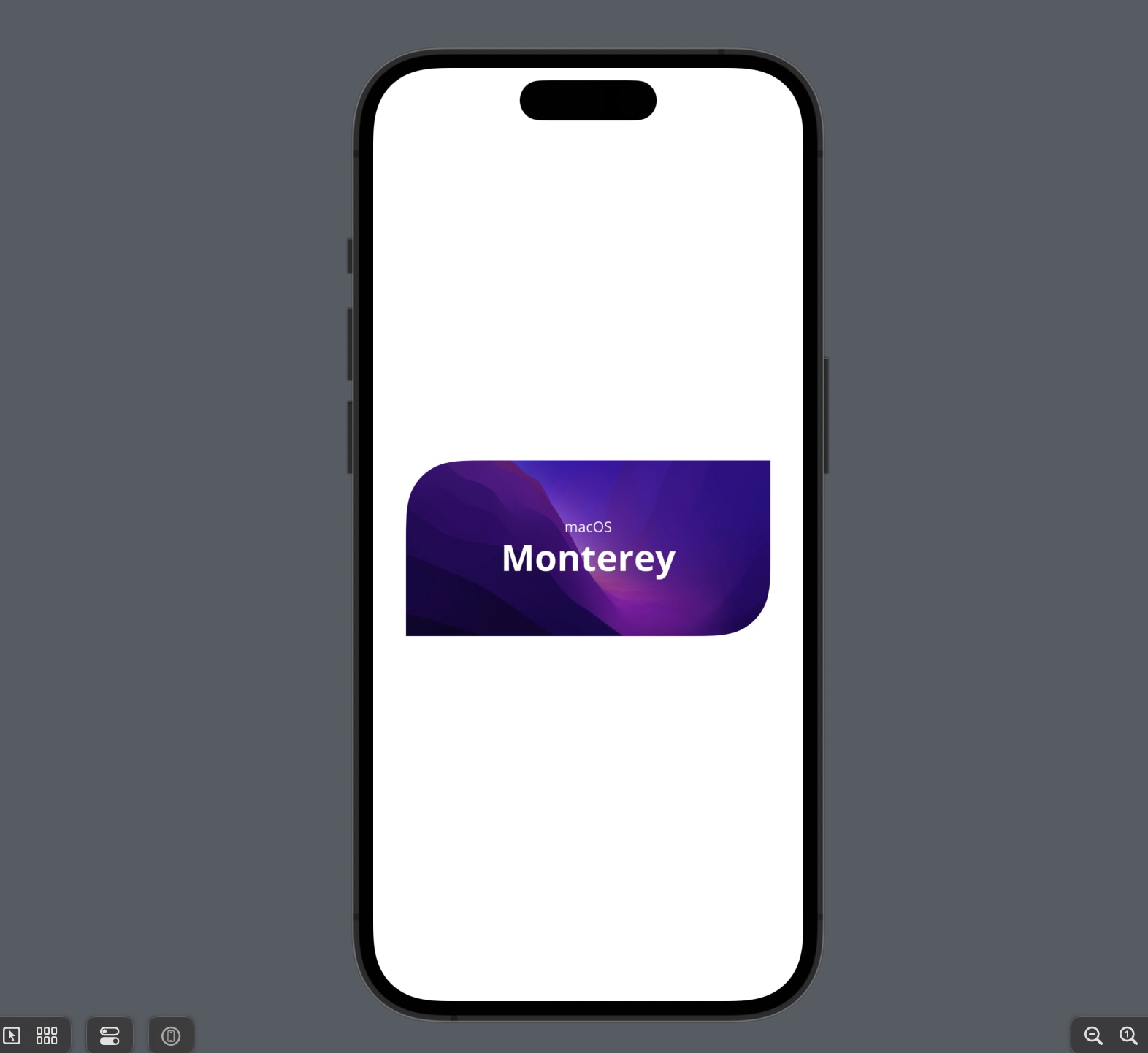
#Controlling Round Corners with background
The background
modifier is typically used to give a background to views that don't have one by default, like Text or Image:
struct ContentView: View {
var body: some View {
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit.")
.foregroundColor(.white)
.padding(30)
.background(
RoundedShape(
radius: 50, corners:
[.topLeft, .bottomLeft]
)
.fill(.orange)
)
}
}
Example of using the background
modifier with custom shape
Don't forget that when you use the background
modifier with a custom shape, you may need to specify the fill color for the shape using the fill
modifier.
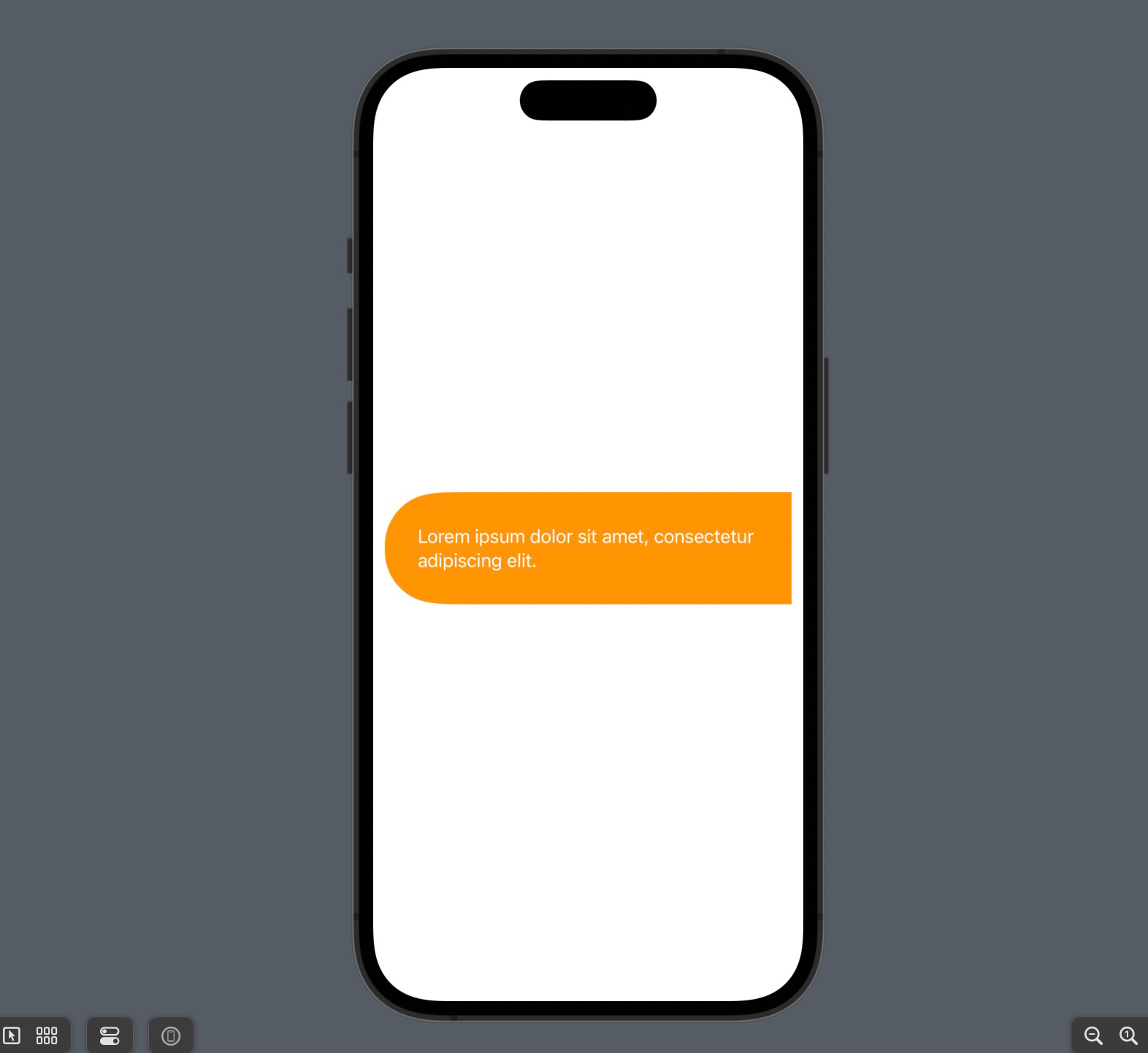
That's all for this tips and tricks article. Give it a try, and we hope this brief guide proves helpful.