Swift Tricks: Accessing App Version Information
Learn how to obtain your app's version and build number using Swift.
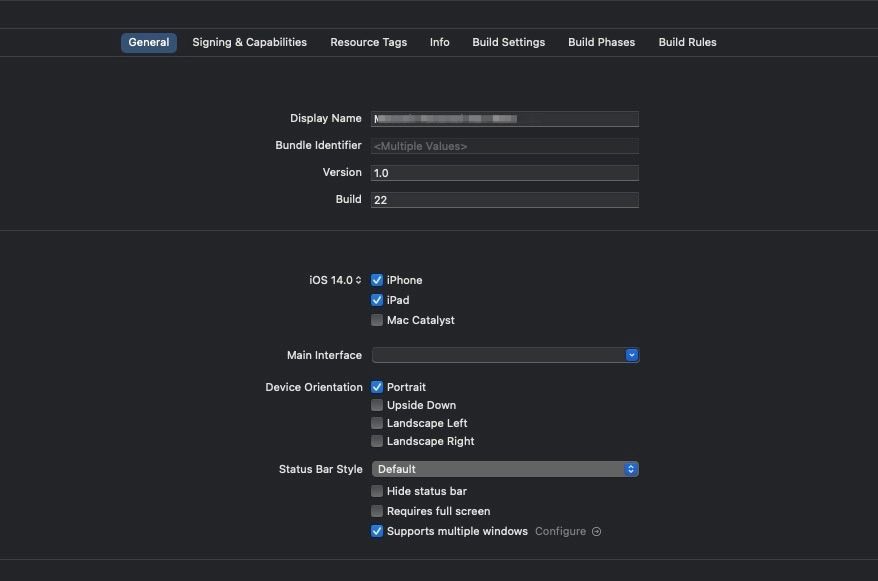
If you're developing an application for iOS or Mac, chances are you're using Xcode. Sometimes, you might want to display the app's version on a particular screen, based on the settings you've configured in Xcode. So, how can you do that?
In a nutshell, the app's version you set in Xcode is stored in the bundle dictionary, with the keys: CFBundleShortVersionString and CFBundleVersion. CFBundleShortVersionString contains the app's version information, while CFBundleVersion contains the build number. To retrieve this information, you simply need to read it from the bundle dictionary as follows.
func get_app_version() -> String {
guard let dictionary = Bundle.main.infoDictionary,
let version: String = dictionary["CFBundleShortVersionString"] as? String,
let build: String = dictionary["CFBundleVersion"] as? String else {
return ""
}
return "v\(version)-\(build)"
}
In the code above, we created a function called get_app_version
to make it easier for use on various screens. It's essential to note that Bundle.main.infoDictionary
is an optional value, meaning it can be nil. Therefore, don't forget to perform unwrapping or check for nil values.
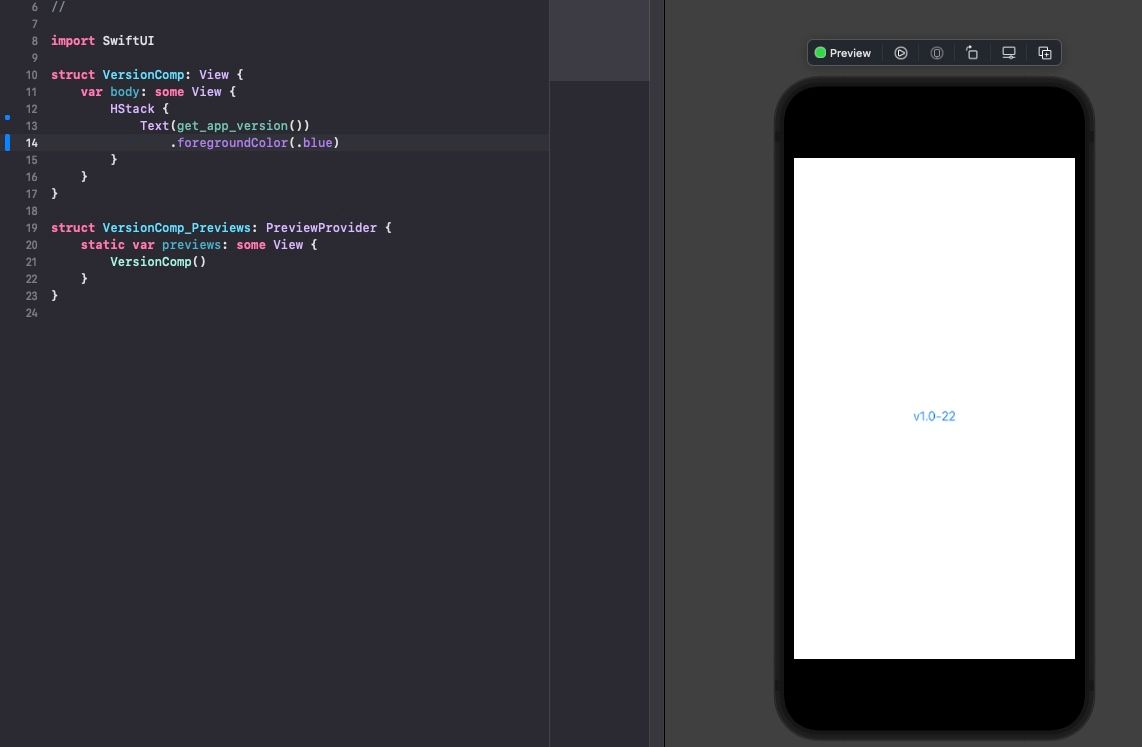
Or if you prefer to write it directly in the view without creating a function, you can do it like this.
import SwiftUI
struct VersionComp: View {
var body: some View {
HStack {
if let dictionary = Bundle.main.infoDictionary,
let version: String = dictionary["CFBundleShortVersionString"] as? String,
let build: String = dictionary["CFBundleVersion"] as? String {
Text("v\(version)-\(build)")
.foregroundColor(.blue)
} else {
Text("no version found")
.foregroundColor(.blue)
}
}
}
}
struct VersionComp_Previews: PreviewProvider {
static var previews: some View {
VersionComp()
}
}
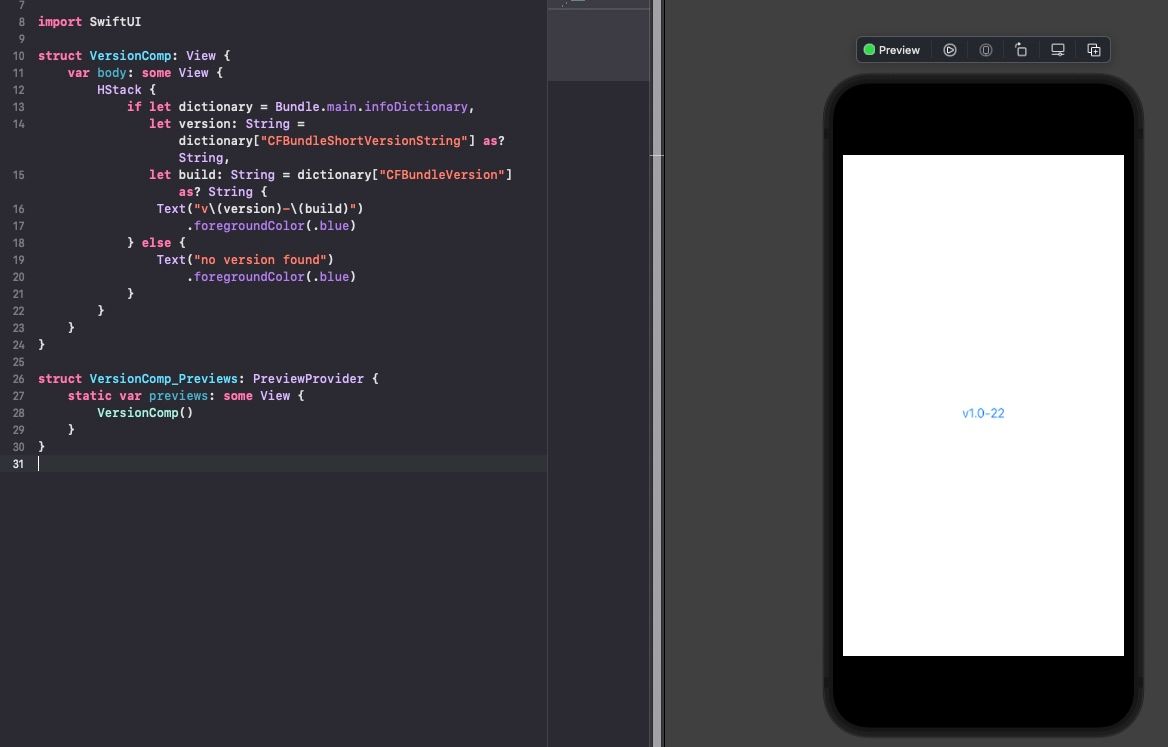
That's all for this quick tip and trick. Give it a try, and hopefully, this little bit is helpful.