Discover How to Convert Hexadecimal Color Codes to SwiftUI Colors
Cara mudah mengubah kode heksadesimal warna menjadi Color di SwiftUI
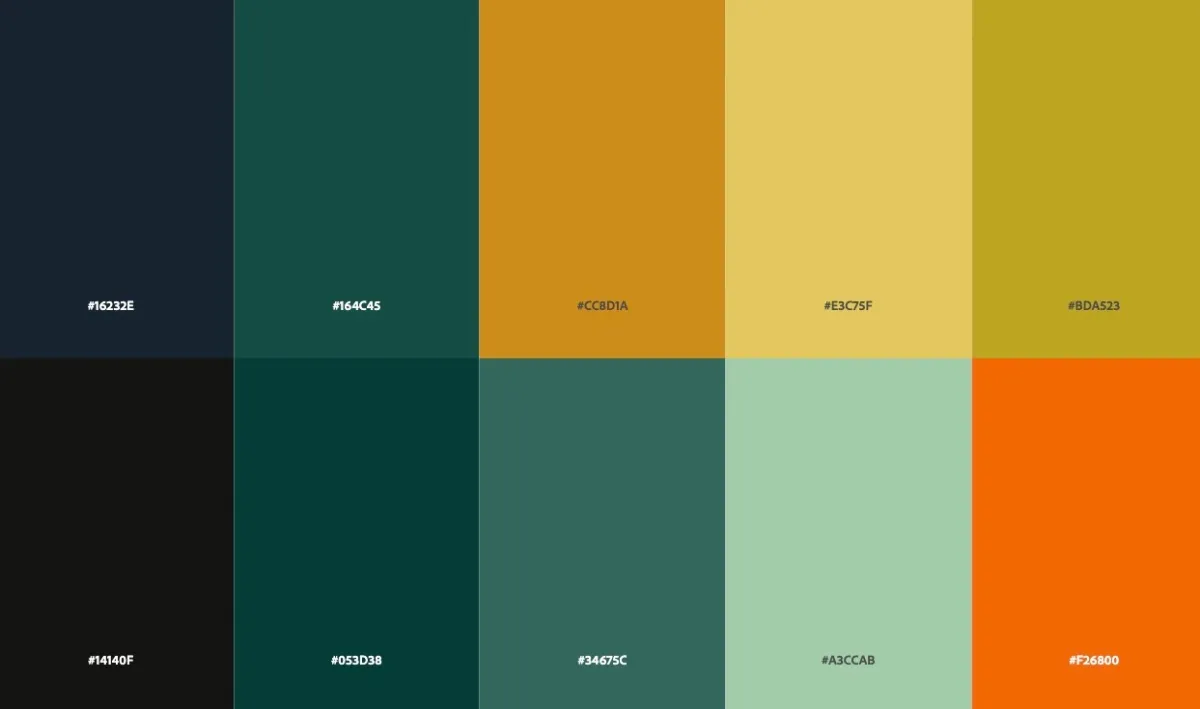
What is a Hexadecimal Code?
Colors can be quickly written in color codes, either in RGB format or hexadecimal codes. For example, the color red can be represented in RGB as rgb(255,0,0) or in hexadecimal as FF0000 or FF0000FF. The hexadecimal code is written in the format RRGGBB or RRGGBBAA. "AA" represents the alpha value or transparency. The value "FF" means the color is fully opaque, while "00" means fully transparent, making the color invisible.
Converting a Hexadecimal String Code
To convert a hexadecimal color string into a Color, you can create an extension of Color as shown in the code below: Converting a hexadecimal string code
extension Color {
init?(hex: String) {
let hex = hex.trimmingCharacters(in: CharacterSet.alphanumerics.inverted)
var int: UInt64 = 0
Scanner(string: hex).scanHexInt64(&int)
let a, r, g, b: UInt64
switch hex.count {
case 6:
(r, g, b, a) = (int >> 16, int >> 8 & 0xFF, int & 0xFF, 255)
case 8:
(r, g, b, a) = (int >> 24, int >> 16 & 0xFF, int >> 8 & 0xFF, int & 0xFF)
default:
return nil
}
self.init(
.sRGB,
red: Double(r) / 255,
green: Double(g) / 255,
blue: Double(b) / 255,
opacity: Double(a) / 255
)
}
}
The code above can be used as follows:
struct ContentView: View {
var body: some View {
VStack {
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Aenean luctus, turpis tristique venenatis lobortis, leo magna posuere magna, ac varius arcu magna at turpis")
.padding()
.background(Color(hex: "F26800"))
}
.padding()
}
}
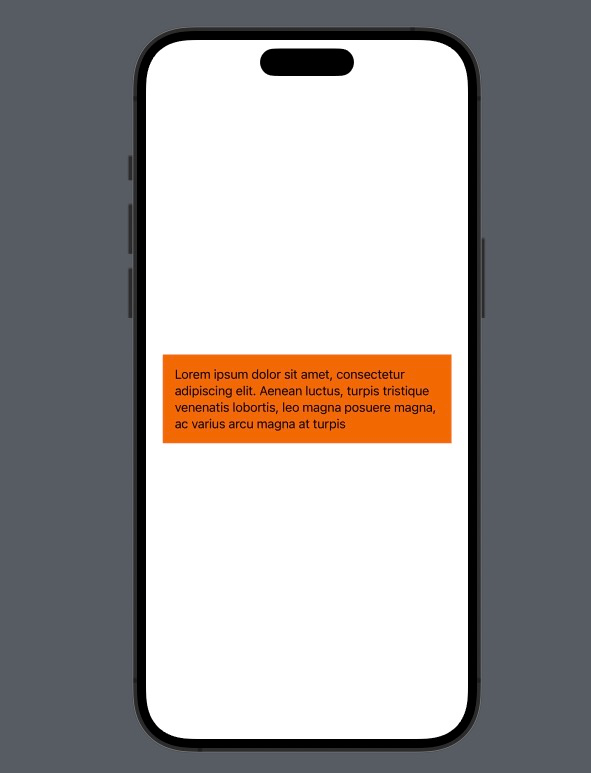
Below is another example of using the Color extension above with input from the user.
Good luck, and hopefully, this small tip will be helpful.