Creating Conditional Modifiers for SwiftUI Views
Implementing conditional modifiers in SwiftUI for easy application of modifiers only when specific conditions are met
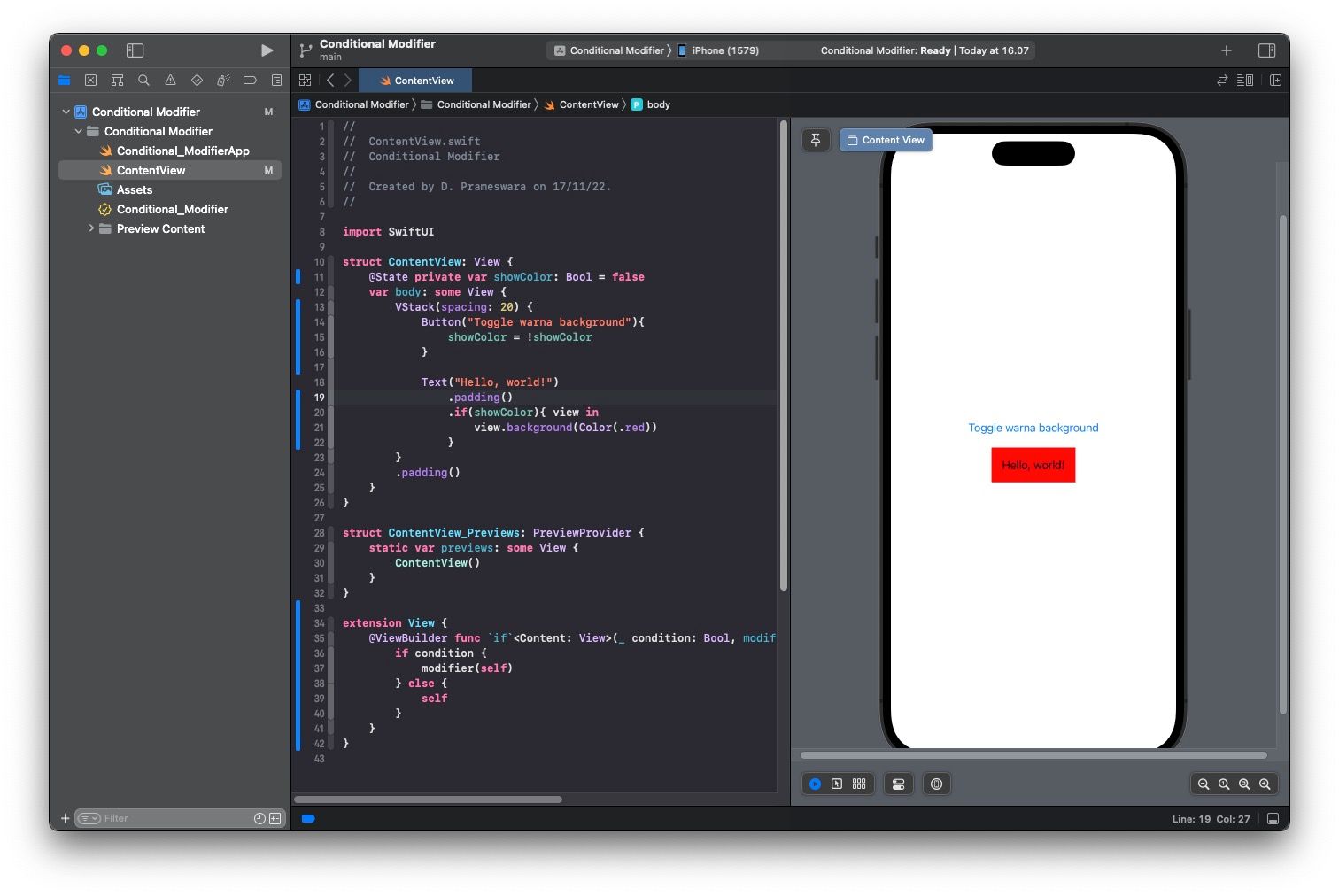
View modifiers are adjustments applied to a view or other view modifiers in SwiftUI to control the style or appearance of a view. For example, you can use .padding
to adjust padding or .background
to set the background of a view.
#View Modifier with a Ternary Condition
Sometimes, you may want to add a modifier to a view only when a specific condition is met. For instance, if a value is true, you want to add a red background; otherwise, you don't want any background. This can generally be achieved by adding a ternary condition, as shown in the example below.
import SwiftUI
struct ContentView: View {
@State private var showColor: Bool = false
var body: some View {
VStack(spacing: 20) {
Button("Toggle warna background"){
showColor = !showColor
}
Text("Hello, world!")
.padding()
.background(Color( showColor ? .red : .clear))
}
.padding()
}
}
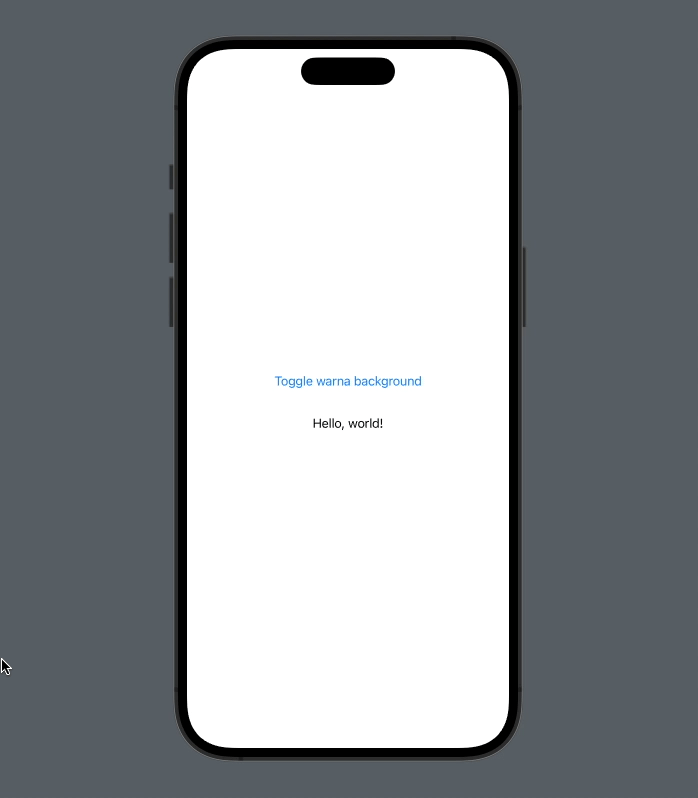
The mentioned ternary condition is .background(Color(showColor ? .red : .clear))
. While this approach is simple, it requires you to specify all conditions explicitly. This falls short of the requirement mentioned above, which is to add a modifier only when specific conditions are met.
#View Modifier with Conditional Modifier
Another solution is to create a conditional modifier. With this approach, you can add a modifier only when specific conditions are met. To achieve this, create an View extension for View as shown below.
extension View {
@ViewBuilder func `if`<Content: View>(_ condition: Bool, modifier: (Self) -> Content) -> some View {
if condition {
modifier(self)
} else {
self
}
}
}
Then, modify the code as follows to use the conditional modifier.
struct ContentView: View {
@State private var showColor: Bool = false
var body: some View {
VStack(spacing: 20) {
Button("Toggle warna background"){
showColor = !showColor
}
Text("Hello, world!")
.padding()
// gunakan conditional modifier
.if(showColor){ view in
view.background(Color(.red))
}
}
.padding()
}
}
Take note of the line .if(showColor) { view in
. Here, we apply the conditional modifier, adding the .background
modifier to the view if the condition is met.
With this conditional modifier, your modifiers are easier to read and understand.
Give it a try, and hopefully, this small improvement will be beneficial.